Roshambo Code Challenge
Here's the CSS, HTML and JavaScriptassignment.
If you want to pass the AWS Certification exams, you need to know a little bit of code. Not a lot, but you do need to know your way around web pages, Java files and modern data exchange formats like YAML and JSON.
- Drag from the left to the right to sort the scrambled Rock-Paper-Scissors code.
- The code will fall into one of three files: index.html, style.css and script.js.
- Save all three files on your desktop.
- Open your index.html file in Chrome and view your page in a web browser!
- You should always lint your code when you get it to work.
- Right-click and choose 'Inspect' in your browser to see your source code and view any console output.
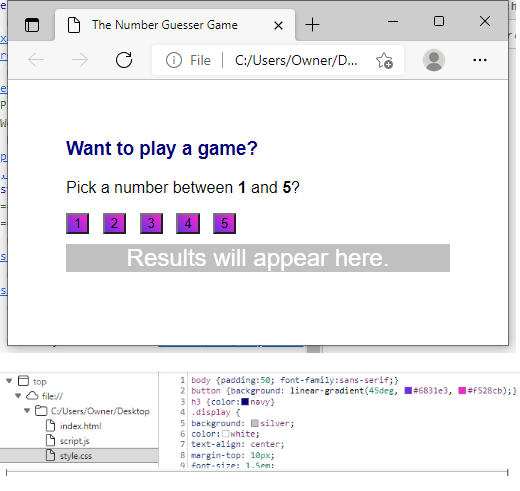
The RAW WebPage
Here's what the latest edition of the number guesser game looks like, with JavaScript, styles and HTML all mixed into one file.
It is an anti-pattern to combine style, structure and script all in one file.
Style, structure and JavaScript should be separated out into .css, .html and .js files for easier management.
<html> <head> <title>The Number Guesser Game</title> <style> body {padding:50; font-family:sans-serif;} button {background: linear-gradient(45deg, #6831e3, #f528cb);} h3 {color:navy} .display { background: silver; color:white; text-align: center; margin-top: 10px; font-size: 1.5em; } </style> </head> <body> <h3>Want to play a game?</h3> <p>Pick a number between <b>1</b> and <b>5</b>? </p> <button onclick="playTheGame('1')"> 1 </button> <button onclick="playTheGame('2')"> 2 </button> <button onclick="playTheGame('3')"> 3 </button> <button onclick="playTheGame('4')"> 4 </button> <button onclick="playTheGame('5')"> 5 </button> <div id="results" class="display">Results will appear here.</div> <script> var magicNumber = Math.floor(Math.random() * 5); playTheGame = function(guess) { var response = "Nope. It's not " + guess; if (guess > magicNumber) { response = response + ". Guess Lower!"; } // close guess lower if if (guess < magicNumber) { response = response + ". Guess Higher!" } // close guess higher if if (guess == magicNumber) { response = "You got it correct!" response += " The number was " + magicNumber + "."; magicNumber = Math.floor(Math.random() * 5); } // end the if document.getElementById('results').innerHTML = response; } // end the method </script> </body> </html>
WARNING: The dragging and dropping function doesn't work great on handhelds.
All Answers Answered
Answers Remain